Posted by Steve on July 12, 2010
A long time ago I did a post on catching JavaScript popups, but have a much better way of catching them.
These JavaScript popups cause trouble as they interrupt the page from fully loading, causing Watir to wait (as the page is waiting), which means the next command in your script will never be reached. Previous work arounds to this were to use watirs built in click_no_wait, but I have that to be extremely temperamental and did not always work depending on which element the click was being performed on.
The new and improved method is to have a completely separate process that runs in the background and is continually checking for JavaScript pop ups. AutoIt commands are used to first locate the pop-up and then depending on what text or title is present in the pop up and different action can be performed on it. Unfortunately the same code cannot be used for both IE and FF due to the fact that the AutoIt controls cannot perform the same actions on IE pop-ups as it can on FF pop-ups. I have included the code for both below:
clickPopupsIE.rb
require 'win32ole'
begin
autoit WIN32OLE.new('AutoItX3.Control')
loop do
autoit.ControlClick("Windows Internet Explorer",'', 'OK')
autoit.ControlClick("Security Information",'', '&Yes')
autoit.ControlClick("Security Alert",'', '&Yes')
autoit.ControlClick("Security Warning",'', 'Yes')
autoit.ControlClick("Message from webpage",'', 'OK')
sleep 1
end
rescue Exception > e
puts e
end
clickPopupsFF.rb
require 'win32ole'
websiteName = "w3schools.com"
begin
autoit = WIN32OLE.new('AutoItX3.Control')
loop do
autoit.winActivate("The page at http://#{websiteName} says:")
autoit.Send("{ENTER}") if(autoit.WinWait("The page at http://#{websiteName} says:",'',2) == 1)
end
rescue Exception => e
puts e
end
These two scripts can then be called from any of your other Watir scripts using the following two functions scripts:
require 'win32/process'
def callPopupKillerFF
$pid = Process.create(:app_name => 'ruby clickPopupsFF.rb', :creation_flags => Process::DETACHED_PROCESS).process_id
end
def callPopupKillerIE
$pid = Process.create(:app_name => 'ruby clickPopupsIE.rb', :creation_flags => Process::DETACHED_PROCESS).process_id
end
def killPopupKiller
Process.kill(9,$pid)
end
As you can see above you do need to require one more ruby gem, ‘win32/process’, this is used to run the popup clicker as a separate process that runs in the background. Once you have those functions in place you can simply call:
callPopupKillerIE #Starts the IE popup killer
#Some watir code that results in a popup#
killPopupKiller #Kills the popup killer process, so that you do not end up with 5 of them running!
Well there you have it, a robust and effective popup killer for both IE and FF. If you have any questions let me know!
–Steve
Posted in IE Automation/Watir/Ruby, Testing | Tagged: AutoIt, Automation, Close, FireFox, IE, JavaScript, Killer, Pop, popup, QA, Ruby, test, Testing, Up, Watir | 12 Comments »
Posted by Steve on December 18, 2009
I recently found a fun bug in the Bevmo (beverages and more, a liquor store in California) website’s search functionality. I was searching for a product from Martini & Rossi, so I simply entered Martini & Rossi into the search field, and clicked ‘Go’ and was surprised when I found myself back on the home page.
At first I was not quite sure what was happening, so as any good tester does, I tried to reproduce the occurrence. So I enter in the same text ‘Martini & Rossi’ and again I am taken to the home page. I decide to isolate the issue, although at this point I am pretty certain that it has to do with the &, so I searched simply the & and found I was taken back to the home page yet again.
At this point I can safely say that the search feature has a bug where if you enter an & you are taken back to the home page, a behaviour that is clearly incorrect. This is a good reminder of why data input is very important when testing fields, it does not take very long and can find some pretty good bugs!
–Steve
Posted in Uncategorized | 1 Comment »
Posted by Steve on November 15, 2009
I recently was in need of a new bluetooth headset, the first one was far too loud on the receiving end up to the point that I was told to just go on mute on a conference call as others could not hear what I was saying at all. The first thing that I did, as often in testing, was to define what my desired end result was. For me what mattered most, above anything else, was the call quality on the receiving end. The other important factors were comfort, and a mute function (as my phone did not have one, and to mute with the conference call system, you had to press *1, something that is not easy while driving).
With the desired qualities in mind, I proceeded to do some research to try and figure out what device what meet my requirements. I found it was easy to narrow down the pool to a couple devices, the Jawbone Prime and the Plantronics Voyager Pro based mainly on editor and personal reviews. Both units were similarly priced, at the time the Jawbone prime was 120$ and the Plantronics Pro was 100$, so they were both upper end devices. This is where I ran into some difficulties; once I was down to the two devices, it was impossible to tell, from reading at least, which device would have the superior call quality. In words it is almost impossible to quantify call quality, often reviewers would say the quality was a four stars, or that it was better than its predecessor. I even found articles comparing the two devices, but I was surprised to discover none of them had any sound clips of what the receiving call sounded like. To me it seemed the only real way to get a feel for how good the call quality was.
This is when I decided move forward and do some testing of my own. I purchased both the Jawbone Prime and the Plantronics Voyager Pro headsets, and I then decided to leave myself a message on my phone, that way I could do a straight comparison of the receiving call quality. I actually ended up leaving messages twice for each device, as the first time I did not have a good idea of what I wanted to test, this was a good example of the importance of having various testing scenarios in mind. I wanted to hear the call quality in a variety of scenarios including, music playing, windows down, air conditioning pointed at face and different voice volume levels. (Unfortunately to hear the tests, you will need to actually download the .wav file, hopefully I can post them on another site that will stream them)
The Plantronics Voyager Pro:
http://www.mediafire.com/file/zuyznjijldm/Plantronics.WAV
The Jawbone Prime:
http://www.mediafire.com/?m5mgwtn3xny
After listening to both clips it was clear that the Plantronics Voyager pro had superior receiving call quality. The Jawbone Prime, was almost perfect when I was not talking, but when I was the background noise started to leak through. Overall comfort was about equivalent for both devices, and the Plantronics Pro had mute functionality where as the Jawbone Prime does not.
I would therefore state that the Plantronics Voyager Pro is the superior device based on my criteria, but both devices performed quite well (especially in comparison to some of the cheaper products). I have had the voyager pro for about 4 months now, and it has continued to perform well. To tie this back to testing, it was interesting because I got to test a product in a non traditional manner and in a realistic setting as a customer. It also reinforced the importance of knowing the needs of the customer when testing, because had my criteria been different a different headset could have come out on top.
–Steve
Posted in Testing | Tagged: Blue, Bluetooth, Comparison, head, headset, Jawbone, Jawbone Prime, Plantronics, product testing, Real world testing, review, set, Tooth, Voyager Pro | 2 Comments »
Posted by Steve on April 23, 2009
A friend recently sent out a screenshot of a quite humourous error message. He was attempting to free up some space on a network drive, by deleting some files, when he received this less than helpful error message:

Error message that appeared when deleting a file
The text in the error message is:
Cannot delete week4: There is not enough free disk space.
Delete one or more files to free disk space, and then try again.
It can be seen that this is a faulty error message, and something else is really afoot; one cannot delete files if one cannot delete files.
An interesting question here is why this error message came up? Is this the actual reason the file cannot be deleted, if so a different error message should be displayed so as not to confuse people. If this is not the cause of the issue, then why did this message get displayed?
–Steve
Posted in Bugs in the wild | Tagged: Bugs, Bugs in the wild, cannot delete file, delete, error, file, funny error, message, not enough disk space, paradox, paradoxical, windows | 1 Comment »
Posted by Steve on April 22, 2009
I ran into this issue when creating my post for importing/exporting individual tables in MySQL. The issue resolves around the principal of WYSIWYG (What you see is what you get).
For one of the MySQL commands it was necessary to have two dashes (or minuses) next to each other, as can be seen in my the post mentioned above. In the WordPress text editor displayed the two dashes properly in both the visual and HTML views, but after I had published the post, the two dashes where displayed (and when copied acted) as a single dash! For example the character in the quotation is seen as two dashes in the editor “–” but as can be clearly seen it is displayed as a single dash.
I tried for a while to resolve the issue, but I ended up just spacing out the dashes, like so “- -“, so that it could be seen that there were actually two dashes.
I feel this falls under a WYSIWYG type issue as in the text editor I was able to see the two dashes properly, but then when the post was published the same thing was not displayed therefore what I was seeing n the editor was not what I got in the post.
–Steve
Posted in Bugs in the wild, Testing, Uncategorized | Tagged: bug, Bugs in the wild, issue, Testing, What You See Is What You Get, wild, wordpress, wysiwyg | 4 Comments »
Posted by Steve on April 21, 2009
In moving databases from development to production it is sometimes necessary to export individual tables so that they can be imported into another database.
Exporting the Table
To export the table run the following command from the command line:
“mysqldump -p – –user=username dbname tableName > tableName.sql”
This will export the tableName to the file tableName.sql.
[NOTE: there should be no space between the two dashes, but I have to write it that way so that it display properly].
Importing the Table
To import the table run the following command from the command line:
mysql -u username -p -D dbname < tableName.sql
The path to the tableName.sql needs to be prepended with the absolute path to that file. At this point the table will be imported into the DB and you are ready to go!
I ran into this issue when attempting to add new tables to my database. I am unable to run the “LOAD DATA INFILE” command, that I had previously used to populate tables, because Webfaction does not give the permission to run the command. Therefore the simplest solution was to export a table from the MySQL database on my personal machine and then import it to the database on the Webfaction server, using the export/import commands seen above.
Hope this helps someone out with exporting individual tables and as always if any clarification is needed or I missed something feel free to let me know.
–Steve
Posted in Uncategorized | Tagged: export, exporting, import, importing, individual, LOAD DATA INFILE, MySQL, one, single, table, tables, webfaction | 3 Comments »
Posted by Steve on April 2, 2009
I have recently had a somewhat frequent recurrence of an error message on my laptop, which is running Windows Vista. The error message can be seen below:
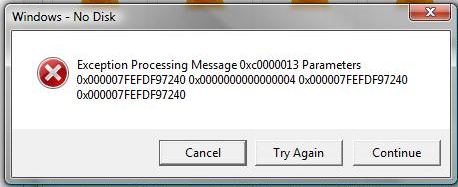
Windows Vista Error Message
The error message popped up (for seemingly no apparent reason), and to make matters worse, when I clicked cancel a new instance of the error message popped up, and I had to close it about 30-40 times before it stopped popping up!
You’ll notice that the first issue with this error message is that it is extremely uninformative and has indecipherable information, something the user should never see. This lack of information made so that I was unable to identify what was causing the error therefore I was not able to stop it from happening (as a quick note, I did not unplug any disks at the time, so it did not have to do with that). Subsequently, since the first incident, this has occurred a few more times with no real pattern as to what is causing it.
A bad error message is good illustrator to what a good error message should be. A good error message should have the following:
- An informative title – A user should know what caused the issue
- Actual error message should provide user with information on how to fix the problem
- Finally it should provide the user with actions that will help fix the problem identify in the message
The only thing the above error message has going for it is that its title gives a clue as to the issue.
To quickly summarize, the goal of an error message is to inform the user that there has been an error, what caused the error, how to fix the error and finally some actions you can take to fix it.
Posted in Testing | Tagged: anatomy, bad error, error, good error, good error message, message, test, Testing, vista, what to put in error message, windows, Windows No Disk, Windows Vista Error | Leave a Comment »
Posted by Steve on April 1, 2009
When I started my web application for my design project I knew absolutely nothing about django, html, css… so basically any and all web technologies. Therefore for a very long time I did not really know the purpose of the action in the form declaration ( <form action=’.’>) or what the ‘.’ actually meant.
It is actually very simple, the ‘ action=”.” ‘ denotes where the form is being submitted (what URL is being called). Specifically, the “.” means that that form will be submitted to the current location (same page), for example if your url was http://mysite.com/search the form would be submitted to http://mysite.com/search.
Now that we know what the action does, it can be used to have two forms on the same page submit to different location. I created a page where a user can either login or register. To have the two forms submit properly I have the following actions for the two forms:
Login Form: <form action=’/accounts/login/’>
Registration Form: <form action=’/register/’>
Now when the login form gets submitted the /accounts/login/ is called and when the registration form gets submitted the data is sent to /register/. An important note is that the action gets performed on the specified location BEFORE the last ‘/’. For example if I had , the action would be performed on the home page and not the register page. Therefore it is important to always have the trailing ‘/’ .
Hopefully this saved someone some trouble getting multiple forms setup.
If you need any clarification, or more details, drop me a line and I’d be more than happy to help.
–Steve
Posted in Uncategorized | Tagged: ".", action, Django, form, form action=".", html, myuwaterloocourses, one page, two forms, web development | 2 Comments »
Posted by Steve on March 24, 2009
I recently took my 4th year Systems Design Engineering project, which is a Django web application, from the development environment into a production environment and had quite a bit of difficulty, that could have been easily avoided if I had a tutorial to follow!
- Hosting & Domain:
The first thing is to either build a web host or purchase hosting from a company. I purchased hosting form Webfaction as they had a one click deployment for a Django application. A domain name should also be purchased, I bought mine from GoDaddy for 10$.
- Getting Setup on Webfaction:
Webfaction takes a little while to setup your account~2-3 hours, when it is complete you will receive an e-mail and you can then login to their control panel. There are some resources that Webfaction offer to help you get setup, the first is a screencast and the other is a text guide.
- Setting up the Database
Once you are in the control panel area there are a few things you’ll want to do. Firstly you will need to add a database (if you have not done so), this can be done by clicking add new databse from the ‘Databases’ drop down menu.
- Adding your domain name
Under domains/websites select the domain link. Click on the add new button, and then enter your domain, for this tutorial we will assume it is ‘mysite.com’. When you add the domain be sure to enter in the subdomains field ‘www’ .
- Setting up the application to serve the static media
The next thing you will want to do is to add Static/CGI/PHP application to your website to serve the static media files (this is highly recommended). To do so go to the applications link under the domains/websites tab. Next click the add new button, name the application ‘media’ and select the app type as ‘Static/CGI/PHP’ and finally click the create button. Now you have to add the application to your domain, to do so click on the ‘websites’ link, and click the edit button for your website. Under subdomains select any that you would like to include, and then under site-apps ensure that you select the media app and set the url/path to ‘/media’. Click update, and you should be taken to the page as seen here:

After site applications have been configured
- Changes to your settings.py file:
There are a few changes that need to be made to the settings.py file:
DEBUG = False MEDIA_ROOT = ‘/home/username/webapps/media/
MEDIA_URL= ‘/media/’
Replace ‘username’ with the username that you selected for your webfaction account, now you are ready to upload your site. You will also need to change your database settings such that they match the new database created by webfaction.
- Uploading your web applicaion
To upload your web application, you will need to upload your ‘mysite’ application to the webfaction server. To do so you will need to download putty (an ssh tool) so that you can ssh into the host, the connection info as well as the username and password are provided by webfaction. Once you are logged in navigate to ‘/home/username/webapps/django/’ and replace the ‘myproject’ folder with your web application folder that you used for development. Once it has been uploaded you will need to tell apache where to locate the project, so naviagte to ‘/home/username/webapps/django/apache2/conf/’ and edit the httpd.conf file. You will need to change th PythonPath and SetEnvPythonPath “[‘/home/username/webapps/django’, ‘/home/username/webapps/django/lib/python2.5′] + sys.path”
SetEnv DJANGO_SETTINGS_MODULE sitename.settings
To setup your static media you will need to and another few lines of code to the same httpd.conf file:
<Location “/media”>
SetHandler None
</Location>
Save the file, and thats all you need to do in the apache folder. One last thing you will need to do is put your existing media into the media directory so that your static files are properly served by apache:
/home/username/webapps/media
- Setting up the Database :
The first thing you will need to do is to get a dump of your current sql database, I personally used mysql, and got a dump with the following line of code: mysqldump -u [username] -p [password] [databasename] > [backupfile.sql] . Now you need to navigate to your project on the webfaction host, /home/username/webapps/django/mysite/ and enter the db shell by entering: python2.5 manage.py dbshell . You should see a mysql command line promp where you can upload your mysql dump: mysql – u user_name -p your_password database_name < file_name.sql. Now you have your database loaded up and you are ready to go.
- Firing it Up:
Everything is ready to go, the last step is to fire up your apache server, to so navigate to /home/username/webapps/django/apache2/ and enter the command bin/stop to stop it in case it was running, and then enter bin/start to start it up.
- Pointing the domain name to the webfaction name servers:
There actualy is one last thing to do, and that is on the domain side of things. You will have to go to the site where you purchased your domain name, and then change the nameservers to those at webfaction, which are as follows:
ns1.webfaction.com
ns2.webfaction.com
ns3.webfaction.com
ns4.webfaction.com
Now you can navigate to http://mysite.com and it should come up, congrats your site is live!
There you have it, from development to production. Also remember if you make any changes to the settings.py file you will have to stop and then restart the apache server. Hopefully this quick tutorial will help you get up and going, and if you have any other questions feel free to ask me!
–Steve
Posted in Fourth Year Project | Tagged: 4th, apache, design, development, Django, Engineering, fourth, MySQL, production, Project, settings.py, systems, waterloo engineering, Web Application, webfaction, year | Leave a Comment »
Posted by Steve on March 18, 2009
I am sure many of you have seen the new 3rd generation iPod shuffle, as seen here. What really caught my attention was this line from the VP of product marketing:
“The new iPod shuffle is the world’s smallest music player and takes a revolutionary approach to how you listen to your music by talking to you, also making it the first iPod shuffle with playlists.”
What catches my attention is that they state that their new ‘revolutionary’ VoiceOver feature; the ‘revolutionary’ VoiceOver feature will tell you the song titles, artists and playlist names. To me the fact that the shuffle talks to you is not a revolutionary change in the way people listen to music.I think they need a little lesson in what constitutes a revolutionary design and what really is an evolutionary design.
The terms evolutionary and revolutionary may seem very similar but there is an important difference, I’ll start by describing both. Evolutionary designs are ones that simply take a design to the next logical step, for example the first iPod nano with video support was an evolutionary design, it brought video to a small portable device but it did not really change how people watch videos. Revolutionary designs are those that fundamentally change how something is done, a classic example of a revolutionary design is the printing press, it fundamentally changed how information was desiminated and possibly led to the prevalence of literacy.
It should be obvious at this point how the new VoiceOver technology is not a revolutionary step in the way that we listen to music. The VoiceOver technology simply provides the same information that a visual display would, just through the auditory channel, thus not really changing how we listen to music.
To me, an example of a revolutionary design for a new music player would be one that could sense our moods and then auto create playlists based on them, or one whose controls could be operated based on our thoughts instead of the current tactile interface. Now obviously I know that these ideas are a little far fetched and currently infeasible, but one can always dream!
———————
Do you agree or disagree with the arguments made above? Let me know, I’d love to hear your thoughts.
Posted in design | Tagged: 3rd Generation, design, evolutionary, iPod, listen to, music, revolutionary, Shuffle, VoiceOver | 3 Comments »